Grade 12 Coding Documentation
Contents
1.Library
2.Emirp
3.FiboString
4.Odius
5.Prime Factors
6.The String
7.Employee
8.Exchange
9.Sorter
10.Pg204
11.SetDemo
12.Hifact
13.RecursiveFibonnaci
14. Program which inputs a positive natural number N and prints the possible consecutive number combinations, which when added give N.
15. Program to print a rectangle with diamond shape with characters, exactly at the center of the rectangle, using an input string
16. Program that takes 7 3 1 6 4 2 5 and makes it 1, 3, 5, 7, 6, 4, 2 after rearranging it, with no extra array
17.Dates
18.ISBN
19.LinkedList
20.Composite Magic Numbers
21.Matrix Mirror
22.Matrix Spiral
23.Square Rotate
24.Array
25.IndexedArray
26.Binary Tree
27.Wondrous Square
28.Evil Numbers
29.Stack
30.Anagrams
31.Queue
1.Library
//calculate charges and fine to be charged on a book borrowed from the library
import java.util.*;
class Library
{
String name, author;
float pric, total_amt;
int d, r;
Library ()
{
name= author= " ";
pric= total_amt= 0.0f;
d= r= 0;
}
void accept ()
{
Scanner scr= new Scanner (System.in);
System.out.println("Enter the name of the book");
name= scr.nextLine();
System.out.println("Enter the name of the author");
author= scr.nextLine();
System.out.println("Enter the price of the book");
pric= scr.nextFloat();
while (pric<0)
{
System.out.println("Invalid input, please try again");
pric= scr.nextFloat();
}
System.out.println("Enter the number of days taken in returning the book");
d= scr.nextInt();
while (d<0)
{
System.out.println("Invalid input, please try again");
d= scr.nextInt();
}
}
void compute ()
{
float amt= (0.02f*pric*d);
d=d-5;
if (d>=1)
{
r=r+(d*2);
if (d>5)
{
r=r+((d-5)*3);
if (d>10)
r=r+((d-10)*5);
}
}
total_amt=amt+(float)r;
}
void show ()
{
System.out.println("Book Name Author Fine Total Amount");
System.out.println("---------------------------------------------------------------------------");
int j=20,i;
System.out.print(name);
for (i=0; i<=(j-name.length()); i++)
{
System.out.print(" ");
}
System.out.print(author);
for (i=0; i<=(j-author.length()); i++)
{
System.out.print(" ");
}
System.out.print(r);
j=20;
while (r>0)
{
r=r/10;
j--;
}
for (i=0; i<=j; i++)
{
System.out.print(" ");
}
System.out.print(total_amt);
}
}
public class LibraryRun
{
public static void main()
{
Library lib=new Library();
lib.accept();
lib.compute();
lib.show();
}
}
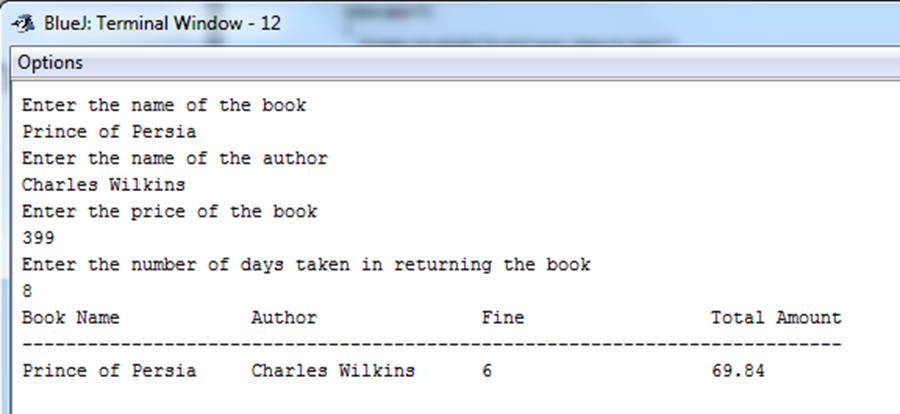
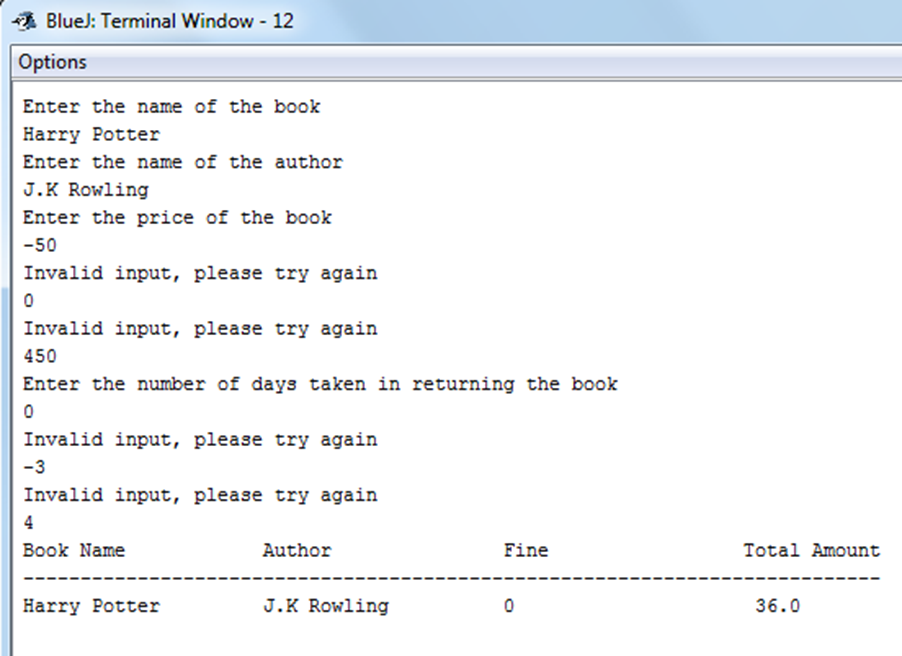
2.Emirp
//checks if a number is prime from left to right and right to left
import java.util.*;
class Emirp
{
int n, rev;
Emirp (int nn)
{
n=nn;
rev=0;
}
int isPrime (int x)
{
int flag= 1;
for (int i=2; i<n/2; i++)
{
if (x%i==0)
flag= -1;
}
return flag;
}
void isEmirp ()
{
int nn;
nn=n;
while (nn>0)
{
rev= (rev*10)+ (nn%10);
nn=nn/10;
}
if (isPrime(n)== 1 && isPrime(n)== 1)
System.out.println ("Number is Emirp");
else
System.out.println ("Number is not Emirp");
}
}
public class EmirpRun
{
public static void main ()
{
Scanner scr=new Scanner (System.in);
int a;
System.out.println("Enter a number:");
a=scr.nextInt();
while (a<0)
{
System.out.println("Invalid Input, please try again");
a=scr.nextInt();
}
Emirp emir=new Emirp (a);
emir.isEmirp();
}
}
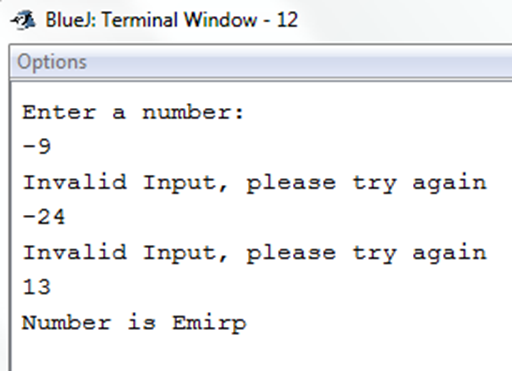
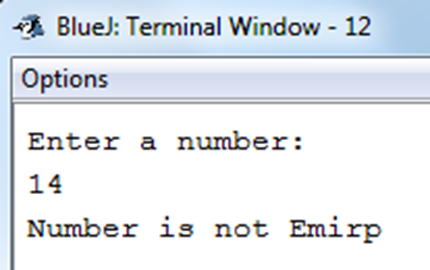
3.FiboString
//prints a Fibonacci sequence of specified length starting with a and b
import java.util.*;
class FiboString
{
String x, y, z;
int n;
FiboString()
{
x="a";
y="b";
z="ba";
}
void accept()
{
Scanner scr=new Scanner (System.in);
System.out.println ("Input the number of terms");
n=scr.nextInt();
while (n<3)
{
System.out.println("Invalid input, please try again");
n=scr.nextInt();
}
}
void generate ()
{
String temp;
System.out.print(x+", "+y+", "+z+", ");
for (int i=0; i<(n-3); i++)
{
temp=z;
z=z+y;
y=temp;
System.out.print(z+", ");
}
}
}
public class FiboStringRun
{
public static void main()
{
FiboString fib=new FiboString();
fib.accept();
fib.generate();
}
}
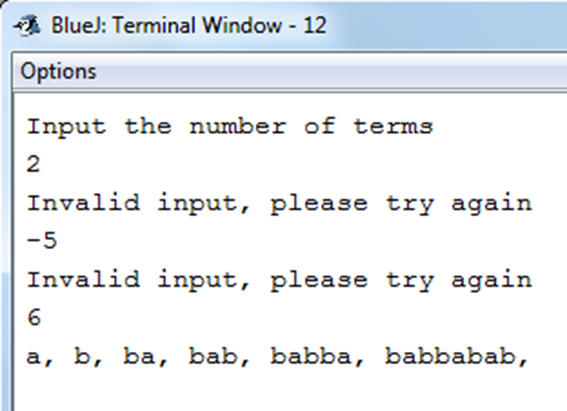
4.Odius
//checks if list of numbers is a nonnegative number that has an odd number of 1s in its binary //expansion
import java.util.*;
class Odious
{
int n, num[]=new int [150];
Odious ()
{
for (int i=0; i<150; i++)
{
num[i]=0;
}
}
Odious (int nx)
{
n=nx;
}
void inputArray()
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter "+n+" elements");
for (int i=0; i<n; i++)
{
num[i]=scr.nextInt();
while (num[i]<0)
{
System.out.println("Invalid Input, please try again");
num[i]=scr.nextInt();
}
}
}
void printOdious()
{
int m, count;
for (int i=0;i<n;i++)
{
m=num[i];
count=0;
while (m>0)
{
if (m%2==1)
count++;
m=m/2;
}
if (count%2==1)
System.out.println("The number "+num[i]+" is odious.");
else
System.out.println("The number "+num[i]+" is not odious.");
}
}
}
public class OdiousRun
{
public static void main ()
{
Scanner scr=new Scanner (System.in);
int n;
System.out.println("Enter size of array");
n=scr.nextInt();
while (n<0)
{
System.out.println("Invalid Input, please try again");
n=scr.nextInt();
}
Odious a=new Odious (n);
a.inputArray();
a.printOdious();
}
}
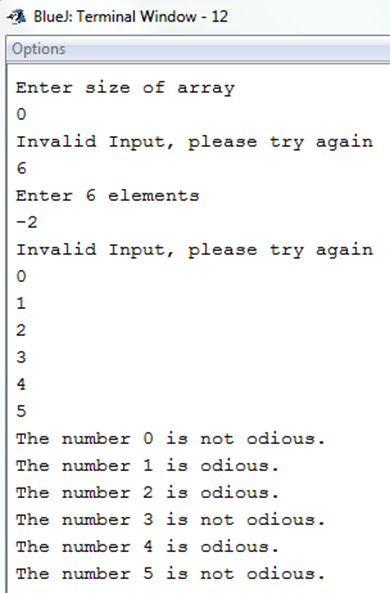
5.Prime Factors
//lists all the prime factors of all the numbers on a list
import java.util.*;
class Prime_Factors
{
int s;
long arr[];
Prime_Factors (int lim)
{
s=lim;
arr=new long [s];
}
void FillArray()
{
Scanner scr=new Scanner (System.in);
for (int i=0;i<s; i++)
{
System.out.println("Enter number in position "+(i+1));
arr[i]=scr.nextLong();
}
System.out.println();
}
void show_PrimeFacts()
{
int count, x;
long n;
for (int i=0;i<s;i++)
{
n=arr[i];
System.out.print(n+": ");
while (n>0)
{
x=2;
while (x<=n)
{
count=0;
for (int j=1; j<=x; j++)
{
if (x%j==0)
count++;
}
if (count<=2 && n%x==0)
{
System.out.print(x+", ");
break;
}
x++;
}
n=n/x;
}
System.out.println();
}
}
}
public class Prime_FactorsRun
{
public static void main ()
{
Scanner scr=new Scanner (System.in);
int n;
System.out.println("Enter size of array");
n=scr.nextInt();
while (n<=0)
{
System.out.println("Invalid input, try again");
n=scr.nextInt();
}
Prime_Factors a=new Prime_Factors (n);
a.FillArray();
a.show_PrimeFacts();
}
}
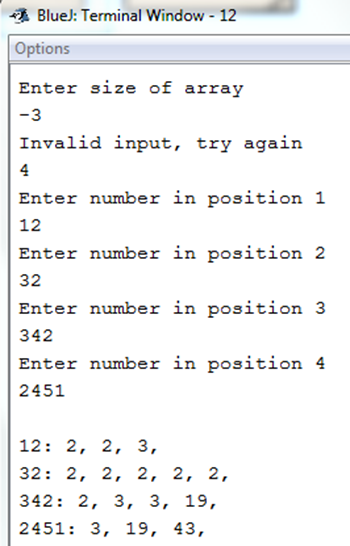
6.The String
//prints number of words and consonants in a given string
import java.util.*;
class TheString
{
int len, wordcount, cons;
String str;
TheString ()
{
len=0;
wordcount=0;
cons=0;
str="";
}
TheString (String ds)
{
str=ds;
}
void countFreq()
{
int i;
wordcount=1;
cons=str.length();
for (i=0; i<str.length(); i++)
{
if (str.charAt(i)==' ')
{
wordcount=wordcount+1;
cons=cons-1;
}
if (Character.isLetter(str.charAt(i))==true)
{
if (str.charAt(i)=='a'||str.charAt(i)=='e'||str.charAt(i)=='i'||str.charAt(i)=='o'||str.charAt(i)=='u'||str.charAt(i)=='A'||str.charAt(i)=='E'||str.charAt(i)=='I'||str.charAt(i)=='O'||str.charAt(i)=='U')
cons=cons-1;
}
}
}
void Display()
{
System.out.println("\""+str+"\" has "+wordcount+" words and "+cons+" consonants");
}
}
public class TheStringRun
{
public static void main (String args[])
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter a String");
String b=scr.nextLine();
TheString a=new TheString (b);
a.countFreq();
a.Display();
}
}
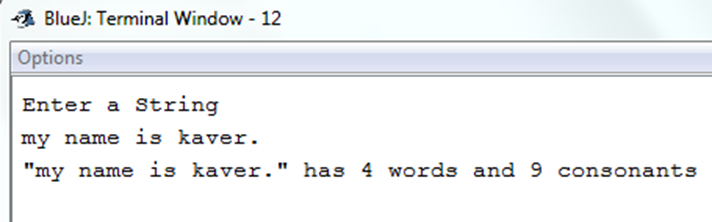
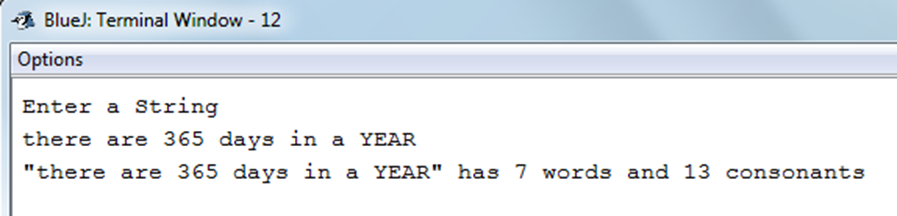
7.Employee
//calculates salary of a person based on their basic pay and employee code
import java.util.*;
class employee
{
String empname;
int empcode;
double basicpay;
employee ()
{
empcode=0;
basicpay=0.0d;
empname="";
}
employee (String n, int p, double q)
{
empname=n;
empcode=p;
basicpay=q;
}
double salarycal ()
{
double sal=0.0d, allow=0.0d;
sal= basicpay + (.3*basicpay) + (0.4*basicpay);
if (empcode<=15 && sal <=15000)
{
allow=(0.2*sal);
if (allow>2500)
allow=2500.0d;
sal=sal+allow;
}
if (empcode>15)
{
allow=1000.0d;
sal=sal+allow;
}
return sal;
}
}
public class employeeRun
{
public static void main (String args[])
{
Scanner scr=new Scanner (System.in);
System.out.println ("Enter the employee name");
String a=scr.nextLine();
System.out.println("Enter employee basic pay");
double d=scr.nextDouble();
while (d<0)
{
System.out.println("Invalid Input, Try again");
d=scr.nextDouble();
}
System.out.println("Enter employee code");
int b=scr.nextInt();
while (b<0)
{
System.out.println("Invalid Input, please try again");
b=scr.nextInt();
}
employee x=new employee (a,b,d);
System.out.println(a+ " has a salary of "+x.salarycal());
}
}
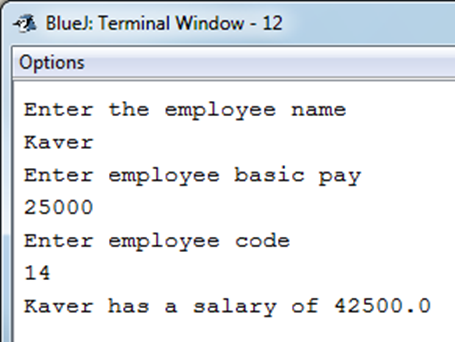
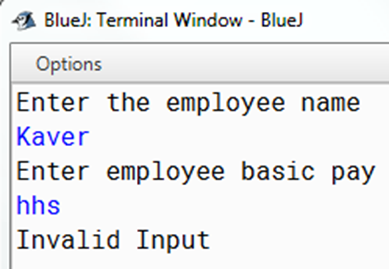
8.Exchange
//switches first and last letter of every word in a sentence
import java.util.*;
class Exchange
{
String sent,rev;
int size;
Exchange()
{
sent=rev="";
size=0;
}
void readsentence()
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter a sentence");
sent=scr.nextLine();
sent=sent.replace('.',' ');
}
void exfirstlast()
{
String a[]=sent.split(" ");
String temp="";
char first;
char last;
for (int i=0;i<a.length;i++)
{
temp=a[i];
if (temp.length()<=1)
{
rev=rev+" "+a[i];
continue;
}
first=temp.charAt(0);
last=temp.charAt(temp.length()-1);
temp=temp.substring(1,(temp.length()-1));
temp=last+temp+first;
a[i]=temp;
rev=rev+" "+a[i];
}
}
void Display()
{
System.out.print("Original sentence: "+sent+".");
System.out.print("New sentence: "+rev);
}
}
public class Exchangerun
{
public static void main (String args[])
{
Exchange b=new Exchange();
b.readsentence();
b.exfirstlast();
b.Display();
}
}
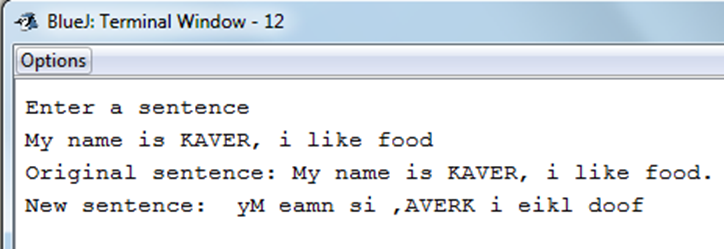
9.Sorter
//sorts an inputed array using insert and selection sort
import java.util.*;
class sorter
{
int arr[]= new int [100];
sorter ()
{
for (int i=0;i<100;i++)
{
arr[i]=0;
}
}
void readlist()
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter 100 integers");
for (int i=0;i<100;i++)
{
arr[i]=scr.nextInt();
}
}
void dispList()
{
for (int i=0;i<100;i++)
{
System.out.print(arr[i]+",");
}
}
void SelectionSort()
{
int j, min, pos, temp;
for (int i=0;i<100;i++)
{
pos=i;
min=arr[i];
for (j=i;j<100;j++)
{
if (arr[j]<min)
{
min=arr[j];
pos=j;
}
}
temp=arr[pos];
arr[pos]=arr[i];
arr[i]=temp;
}
}
void InsSort()
{
int i, j, temp;
for (i = 1; i<50; i++)
{
j = i;
temp = arr[i];
while (j > 0 && temp > arr[j-1])
{
arr[j] = arr[j-1];
j=j-1;
}
arr[j] = temp;
}
}
}
public class sorterRun
{
public static void main(String args[])
{
sorter sor=new sorter ();
sor.readlist();
System.out.println();
System.out.println("Insertion sort: ");
sor.InsSort();
sor.dispList();
System.out.println();
System.out.println("Selection sort:");
sor.SelectionSort();
sor.dispList();
}
}
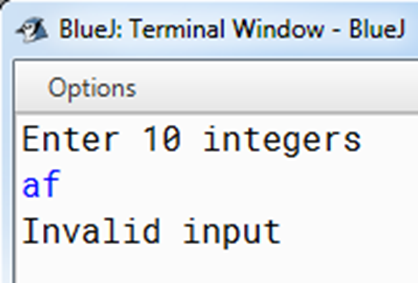
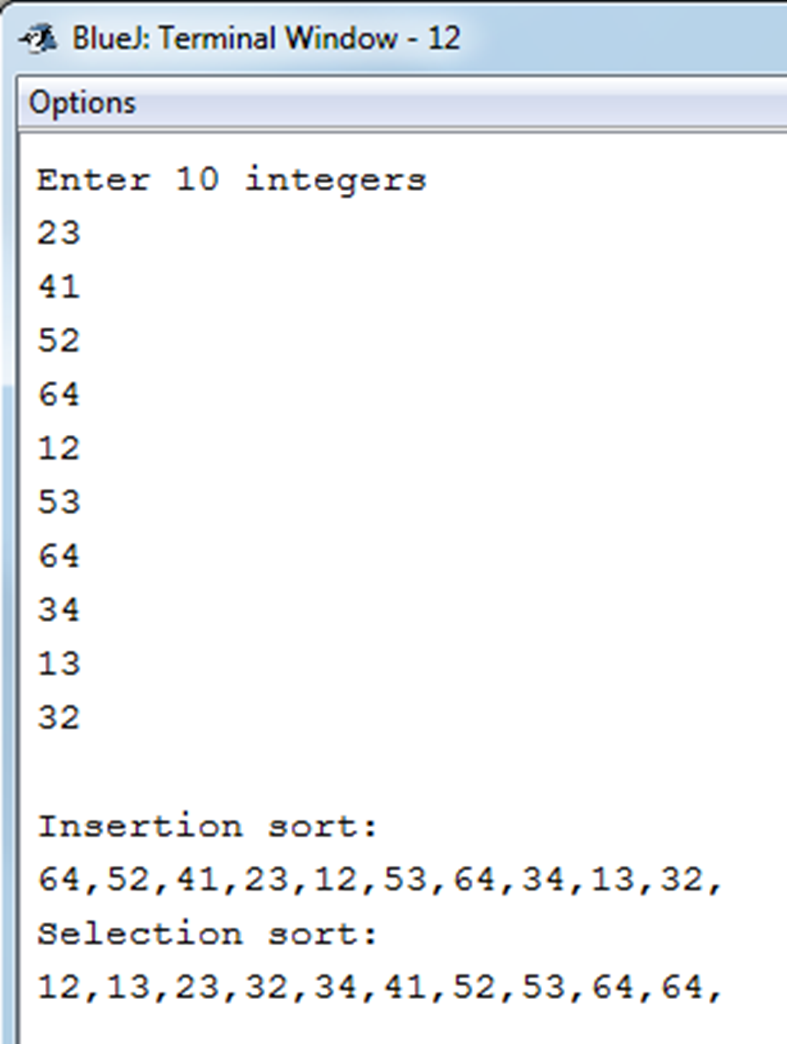
//to find a number that is greater than the 1st inputted number whose digits add up to the second inputted number
import java.util.*;
public class Pg204
{
public static void main (String args[])
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter Integer M which is greater than 100 and less than 10000");
long m=scr.nextLong();
while (m>10000||m<100)
{
System.out.println("Invalid Input, try again");
m=scr.nextInt();
}
System.out.println("Enter Integer N which is greater than 0 and less than 100");
long n=scr.nextLong();
while (n>100 || n<0)
{
System.out.println("Invalid Input, try again");
n=scr.nextInt();
}
long sum=0, count=0, x=m, i=m;
while (sum!=n)
{
sum=0;
x=i;
count=0;
while (x>0)
{
sum=sum+(x%10);
x=x/10;
count++;
}
i++;
}
System.out.println();
System.out.println("The required number= "+(i-1));
System.out.println("Total number of digits="+count);
}
}
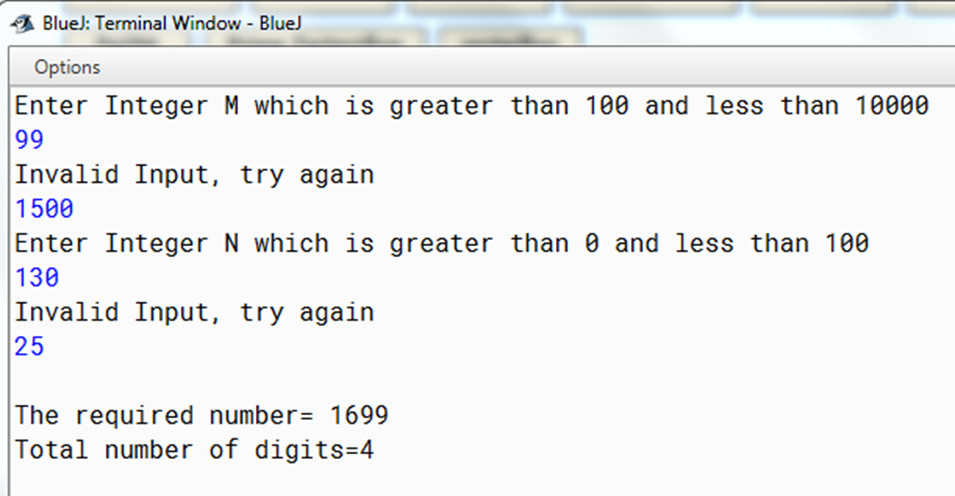
11.SetDemo
//find union and intersection of two sets
import java.util.*;
public class SetRun
{
public static void main(String args[])
{
try{
Set a=new Set(5);
a.readElements();
Set b=new Set(5);
b.readElements();
Set c=new Set (10);
System.out.println("Union:");
c=a.union(b);
int y, i;
for (i=0; i<c.arr.length; i++)
{
y=c.arr[i];
for (int j=i+1; j<c.arr.length-1; j++)
{
if (c.arr[j]==y)
c.arr[j]=0;
}
}
c.displayElements();
System.out.println();
System.out.println("Intersection:");
c=a.intersection(b);
c.displayElements();
System.out.println();
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
class Set
{
int arr[];
int N;
Set (int nn)
{
N=nn;
if (N<=50)
arr=new int[N];
else N=50;
}
void readElements()
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter Array");
for (int i=0;i<this.getSize();i++)
{
arr[i]=scr.nextInt();
}
}
void displayElements()
{
for(int i=0;i<this.getSize();i++)
{
if (arr[i]!=0)
System.out.print (arr[i]+",");
}
}
int getSize()
{
return N;
}
int has(int ele)
{
for (int i=0; i<this.getSize(); i++)
{
if (arr[i]==ele)
return 1;
}
return 0;
}
Set intersection(Set d)
{
for(int i=0;i<this.getSize();i++)
{
if ((this.has(d.arr[i]))==0)
d.arr[i]=0;
}
return d;
}
Set union(Set d)
{
int p=0,q=0;
Set f=new Set ((this.getSize())+d.getSize());
for(int i=0;i<(this.getSize()+d.getSize());i++)
{
if (i%2==0)
{
if (p>=this.getSize())
{
f.arr[i]=d.arr[q];
q=q+1;
continue;
}
f.arr[i]=arr[p];
p=p+1;
}
else
{
f.arr[i]=d.arr[q];
q=q+1;
}
}
return f;
}
}
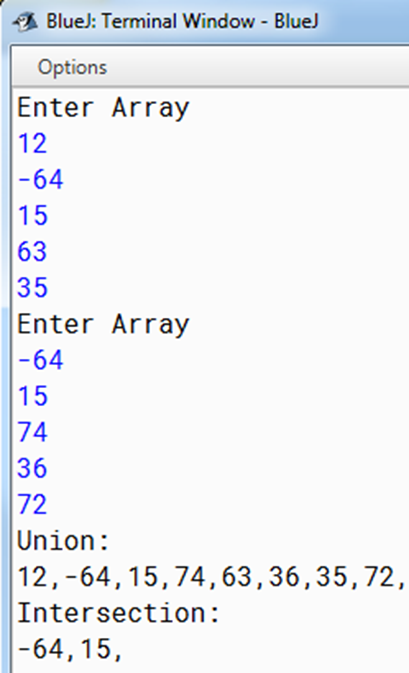
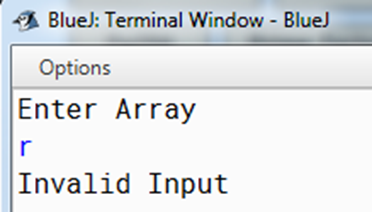
12.Hifact
//find hcf and lcm recursively
import java.util.*;
class Hifact
{
int a,b,hcf,lcm;
Hifact()
{
a=b=hcf=lcm=0;
}
void getdata()
{
System.out.println("Enter two numbers");
Scanner scr=new Scanner (System.in);
a=scr.nextInt();
b=scr.nextInt();
}
void change()
{
int c;
if (b>a)
{
c=b;
b=a;
a=c;
}
}
int rechcf(int l, int m)
{
if (a%m==0&&b%m==0)
{
hcf=m;
return hcf;
}
else
return rechcf(l,m-1);
}
int fn_lcm(int l, int m, int n)
{
return (l*m)/n;
}
void result()
{
change ();
hcf=rechcf (a,b);
lcm=fn_lcm (a,b,hcf);
}
}
public class HifactRun
{
public static void main (String args[])
{
Hifact h=new Hifact();
try
{
h.getdata();
h.result();
System.out.println("hcf="+h.hcf);
System.out.println("lcm="+h.lcm);
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
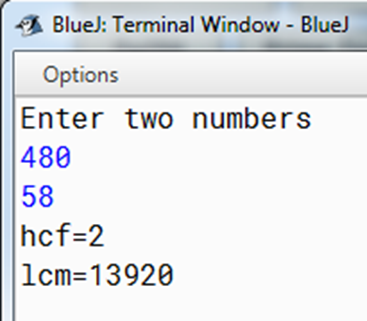
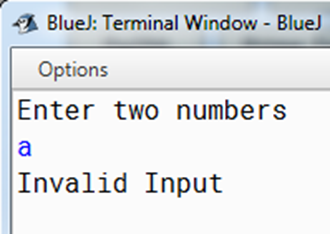
13.RecursiveFibonnaci
//prints fibonnaci series of given length recursively
import java.util.*;
class RecurFibb
{
int a,b,c,limit;
RecurFibb()
{
a=0;
b=1;
c=1;
}
void input()
{
System.out.println("Enter length of series");
Scanner scr=new Scanner (System.in);
limit=scr.nextInt();
}
int fib(int n)
{
if (n==1)
return 1;
else
{
b=fib(n-1);
c=a+b;
a=b;
return c;
}
}
void generatefibseries()
{
for (int i=1;i<=limit;i++)
{
System.out.println(fib(i));
a=0;
b=1;
c=1;
}
}
}
public class RecurFibbRun
{
public static void main(String args[])
{
RecurFibb a=new RecurFibb();
a.input();
a.generatefibseries();
}
}
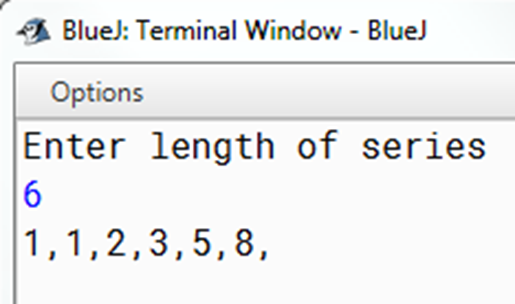
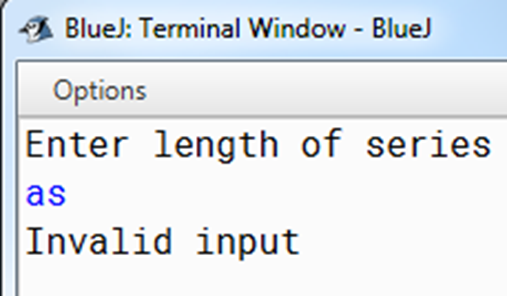
14. Program which inputs a positive natural number N and prints the possible consecutive number combinations, which when added give N.
import java.util.*;
class MidTermPracQ1
{
public static void main (String args[])
{
Scanner scr=new Scanner (System.in);
int N,i,j,x,sum=0;
System.out.println("Input a positive natural number");
N=scr.nextInt();
if (N<=0)//checking if N is a positive natural number and showing error if not
{
System.out.println("Wrong input, try again");
N=scr.nextInt();
}
System.out.println("INPUT");
System.out.println(" N="+N);
x=N/2;
System.out.println("OUTPUT");
if ((x+(x+1))==N)
System.out.println(" "+x+" "+(x+1)); //checking if sum of N/2-1 and N is equal
if ((x+(x-1))==N) //to N
System.out.println(" "+(x-1)+" "+x);
System.out.print(" ");
for (i=1;i<(x-1);i++)//incrementing from 1 to N to check starting point of consecutive sum series
{
sum=0;
j=i;
while (sum<N)//checking if consecutive sum series can be made from this starting point
{
sum=sum+j;
j++;
}
if (sum==N)
{
sum=0;
j=i;
while (sum<N)//checking if consecutive sum series can be made from this starting point
{
sum=sum+j;
System.out.print(j+" ");
j++;
}
System.out.println();
System.out.print(" ");
}
}
}
}
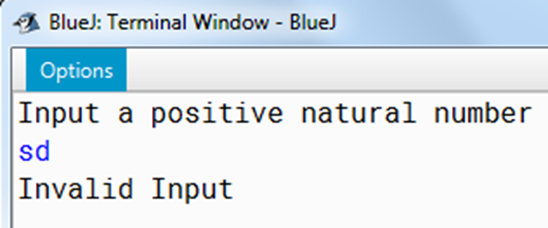
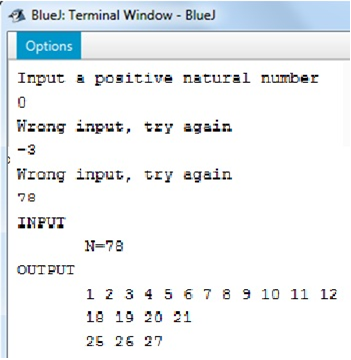
15. Program to print a rectangle with diamond shape with characters, exactly at the center of the rectangle, using an input string
import java.util.*;
public class MidTermPracQ2
{
public static void main (String args[])
{
Scanner obj=new Scanner (System.in);
String s;
int i, j=0, space, k;
System.out.println("Enter a String");
s=obj.next();
while (s.length()<20&&s.length()%2==0)//checking if input is valid
{
System.out.println("Wrong input, please try again");
s=obj.next();
}
for (i=0;i<((s.length()/2)+1);i++)
{
space=s.length()-j;
for (k=0;k< space;k++)
System.out.print(" ");
System.out.print(s.substring(((s.length()/2)-j),((s.length()/2)+j+1)));
j++;
System.out.println();
}
j=s.length()/2;
for (i=0;i<((s.length()/2)+1);i++)
{
space=s.length()-j;
for (k=0;k< space;k++)
System.out.print(" ");
System.out.print(s.substring(((s.length()/2)-j),((s.length()/2)+j+1)));
j--;
System.out.println();
}
}
}
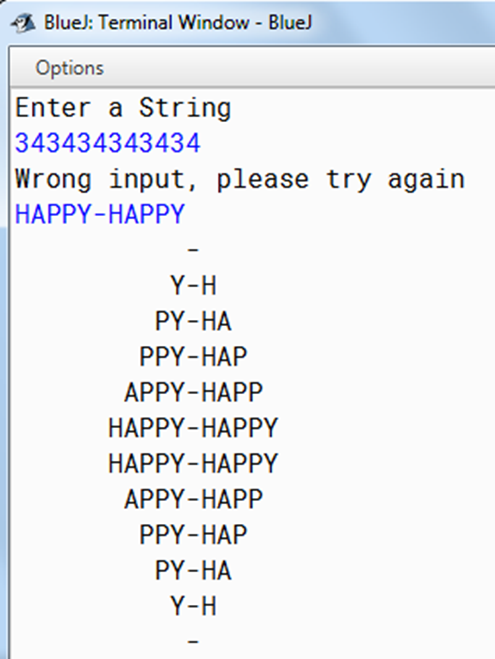
16. Program that takes 7 3 1 6 4 2 5 and makes it 1, 3, 5, 7, 6, 4, 2 after rearranging it, with no extra array
import java.util.*;
class MidTermPracQ3
{
public static void main (String args [])
{
System.out.println("Input :");
System.out.println();
int n, i, max=0, m=0, temp, j, l=1, k, r=1, u;
boolean c=true;
Scanner scr=new Scanner (System.in);
System.out.print("Give the number of Integers:");
n=scr.nextInt();
int a[]=new int [n];
for (i=1;i<=n;i++)
{
System.out.print("Give Integer "+i+":");
a[i-1]=scr.nextInt();
}
System.out.println();
System.out.println("Output:");
System.out.println("Original array");
System.out.println();
for (i=0;i<n;i++)
{
System.out.print(a[i]+" ");
}
for (i=0;i<n;i++)
{
if (a[i]>max)
{
max=a[i];
m=i;
}
}
if (n%2!=0)
i=n/2;
else
i=n/2-1;
k=i;
temp=a[i];
a[i]=a[m];
a[m]=temp;
m=a[i];
u=0;
for (i=0;i<n-1;i++)
{
max=0;
for (j=0;j<n;j++)
{
if (a[j]>max&&a[j]<m)
{
max=a[j];
u=j;
}
}
m=a[u];
if (c==true)
{
temp=a[k+r];
a[(k+r)]=a[u];
a[u]=temp;
r=r+1;
}
else
{
temp=a[k-l];
a[(k-l)]=a[u];
a[u]=temp;
l=l+1;
}
if (c==true)
c=false;
else
c=true;
}
System.out.println();
System.out.println();
System.out.println("Rearranged array");
for (i=0;i<n;i++)
{
System.out.print(a[i]+" ");
}
}
}
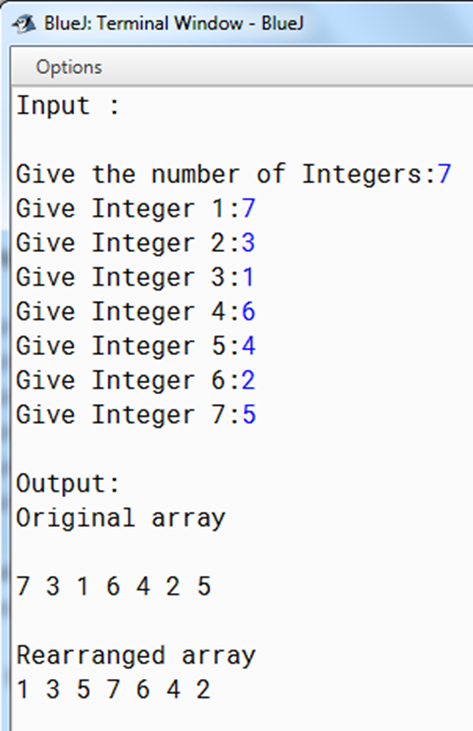
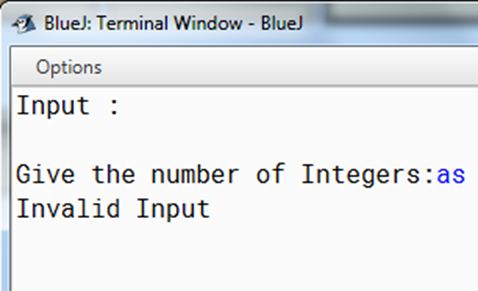
//find number of days between two dates
import java.io.*;
public class July24 {
public static void main (String args []) throws IOException
{
BufferedReader br=new BufferedReader (new InputStreamReader(System.in));
int y, m, d, y1, m1, d1, sum=0, z=0;
System.out.println("Please enter first Date in 2 digits");
d=Integer.parseInt(br.readLine());
System.out.println("Please enter first Month in 2 digits");
m=Integer.parseInt(br.readLine());
System.out.println("Please enter first Year in 4 digits");
y=Integer.parseInt(br.readLine());
System.out.println("Please enter second Day in 2 digits");
d1=Integer.parseInt(br.readLine());
System.out.println("Please enter second Month in 2 digits");
m1=Integer.parseInt(br.readLine());
System.out.println("Please enter second Year in 4 digits");
y1=Integer.parseInt(br.readLine());
if (m1==2)
{
if(y1%100!=0&&y1%4==0||y1%400==0)
{
if (d1>29)
System.exit(0);
}
else
{
if (d1<28)
System.exit(0);
}
}
else
if (y1>y) // swapping the day, month and year to move the bigger date to d, m, y
{
z=d1;
d1=d;
d=z;
z=m1;
m1=m;
m=z;
z=y1;
y1=y;
y=z;
}
if (d1>d)
{
if (m1==2)
{
if(y%100!=0&&y%4==0||y%400==0)
{
sum=sum+(29-d1)+d;
m1=m1+1;
}
else
{
sum=sum+(28-d1)+d;
m1=m1+1;
}
}
if (m1==1||m1==3||m1==5||m1==7||m1==8||m1==10||m1==12)
{
sum=sum+(31- d1)+d;
m1=m1+1;
}
else
{
sum=sum+(30-d1)+d;
m1=m1+1;
}
d=d1;
}
else
{
sum=sum+(d-d1);
d=d1;
}
//System.out.println(sum+" "+m);
for(;m1!=m;m1++)
{
if (m1==13)
{
m1=1;
y1=y1+1;
}
if (m1==2)
{
if(y%100!=0&&y%4==0||y%400==0)
sum=sum+29;
else
sum=sum+28;
}
else
{
if (m1==1||m1==3||m1==5||m1==7||m1==8||m1==10||m1==12)
sum=sum+31;
else
sum=sum+30;
}
//System.out.println(m1);
}
//System.out.println("---------------");
for (;y1!=y; y1++)
{
if(y1%100!=0&&y1%4==0||y1%400==0)
sum=sum+366;
else
sum=sum+365;
//System.out.println(y1);
}
System.out.println("Number of days= "+sum);
}
}
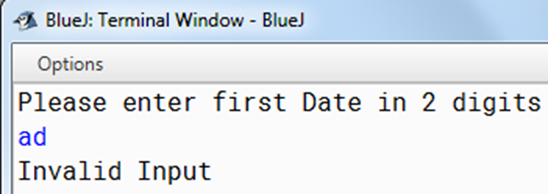
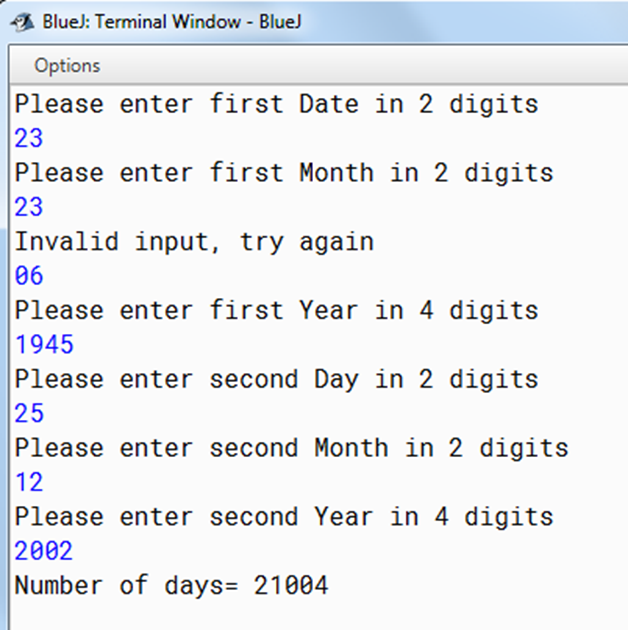
18.ISBN
// Java program to check if
// a given ISBN isvalid or not
import java.util.*;
class ISBN
{
static boolean isValidISBN(String isbn)
{
// length must be 10
int n = isbn.length();
if (n != 10)
return false;
// Computing weighted sum
// of first 9 digits
int sum = 0;
for (int i = 0; i < 9; i++)
{
int digit = isbn.charAt(i) - '0';
if (0 > digit || 9 < digit)
return false;
sum += (digit * (10 - i));
}
// Checking last digit.
char last = isbn.charAt(9);
if (last != 'X' && (last < '0' ||
last > '9'))
return false;
// If last digit is 'X', add 10
// to sum, else add its value
sum += ((last == 'X') ? 10 : (last - '0'));
// Return true if weighted sum
// of digits is divisible by 11.
return (sum % 11 == 0);
}
public static void main(String[] args)
{
try
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter an ISBN code");
String a=scr.nextLine();
if (isValidISBN(a))
System.out.print("Valid");
else
System.out.print("Invalid");
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
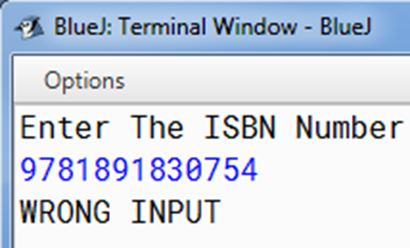
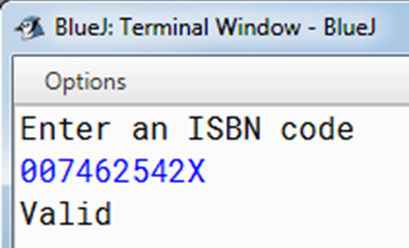
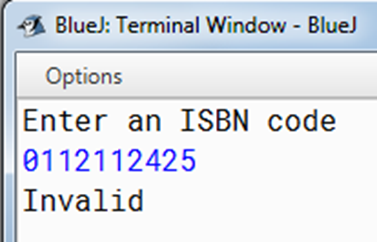
//add nodes, delete nodes, split list, and merge lists.
import java.util.*;
class LinkedList
{
Node start;
LinkedList()
{
start=null;
}
void addNode(String in)
{
Node n=new Node();
n.name=in;
if (start == null)
{
start=n;
}
else
{
for (Node temp=start;temp!=null;temp=temp.ptr)
{
if (temp==start&&temp.name.compareTo(n.name)>0)
{
n.ptr=start;
start=n;
break;
}
if (temp.ptr == null)
{
temp.ptr=n;
break;
}
if (temp.name.compareTo(n.name)<0&&temp.ptr.name.compareTo(n.name)>0)
{
n.ptr=temp.ptr;
temp.ptr=n;
break;
}
if (temp.name.compareTo(n.name)==0)
{
n.ptr=temp.ptr;
temp.ptr=n;
break;
}
}
}
}
void print()
{
for (Node temp=start;temp!=null;temp=temp.ptr)
{
System.out.println(temp.name);
}
}
void delete (String na)
{
if (start.name.equals(na))
{
start=start.ptr;
return;
}
for (Node temp=start;temp!=null;temp=temp.ptr)
{
if (temp.ptr.name.equals(na))
{
temp.ptr=temp.ptr.ptr;
break;
}
}
}
void delete (int na)
{
if (na==1)
{
start=start.ptr;
return;
}
for (Node temp=start;temp!=null;temp=temp.ptr)
{
na--;
if (na==1)
{
temp.ptr=temp.ptr.ptr;
break;
}
}
}
void MergeList(LinkedList x)
{
Scanner scr=new Scanner (System.in);
System.out.println("1.Merge ordered");
System.out.println("2.Merge unordered");
System.out.println("Any other number to go back");
int choice=scr.nextInt();
scr.nextLine();
switch(choice)
{
case 1:
Node temp;
for (temp=start;temp.ptr!=null;temp=temp.ptr);
temp.ptr=x.start;
x.start=null;
break;
case 2:
for (temp=x.start;temp.ptr!=null;temp=temp.ptr)
{
addNode(temp.name);
}
break;
default:
}
}
void SplitList(LinkedList x)
{
Scanner scr=new Scanner (System.in);
boolean flag;
Node del=null;
int count;
System.out.println("How to split the list?");
System.out.println("1.After a certain name");
System.out.println("2.After a certain position");
System.out.println("3.Alternate values in each list");
System.out.println("Any other number to go back");
int choice=scr.nextInt();
scr.nextLine();
switch (choice)
{
case 1:
flag=false;
System.out.println("Enter name to split after");
String nam=scr.nextLine();
for (Node temp=start;temp!=null;temp=temp.ptr)
{
if (flag==true)
{
x.addNode(temp.name);
}
if (temp.name.equals(nam))
{
flag=true;
del=temp;
}
}
del.ptr=null;
break;
case 2:
flag=false;
System.out.println("Enter position to split after");
count=scr.nextInt();
for (Node temp=start;temp!=null;temp=temp.ptr)
{
if (flag==true)
{
x.addNode(temp.name);
}
if (count==1)
{
flag=true;
del=temp;
count=-1;
}
else
count--;
}
del.ptr=null;
break;
case 3:
count=0;
for (Node temp=start;temp!=null;temp=temp.ptr)
{
count++;
if(count%2==0)
{
x.addNode(temp.name);
delete(temp.name);
}
else
continue;
}
break;
default:
}
}
}
public class linkedMain
{
public static void main(String args[])
{
Scanner scr=new Scanner (System.in);
int i, choi=1; String y;
LinkedList ll=new LinkedList();
LinkedList ll2=new LinkedList();
do
{
System.out.println("1.Add Node");
System.out.println("2.Delete Node by name");
System.out.println("3.Delete Node by position");
System.out.println("4.Display List 1");
System.out.println("5.Display List 2");
System.out.println("6.Split List");
System.out.println("7.Merge List");
System.out.println("Any other number to stop");
choi=scr.nextInt();
scr.nextLine();
switch (choi)
{
case 1:
System.out.println("Enter 5 names");
for (i=1;i<=5;i++)
{
y=scr.nextLine();
ll.addNode(y);
}
break;
case 2:
System.out.println("Enter name to delete");
ll.delete(scr.nextLine());
break;
case 3:
System.out.println("Enter position to delete");
ll.delete(scr.nextInt());
break;
case 4:
ll.print();
break;
case 5:
ll2.print();
break;
case 6:
ll.SplitList(ll2);
break;
case 7:
ll.MergeList(ll2);
break;
default:
System.out.println("Bye!");
}
}while(choi<8);
}
}
class Node
{
String name;
Node ptr;
Node()
{
name="";
ptr=null;
}
}
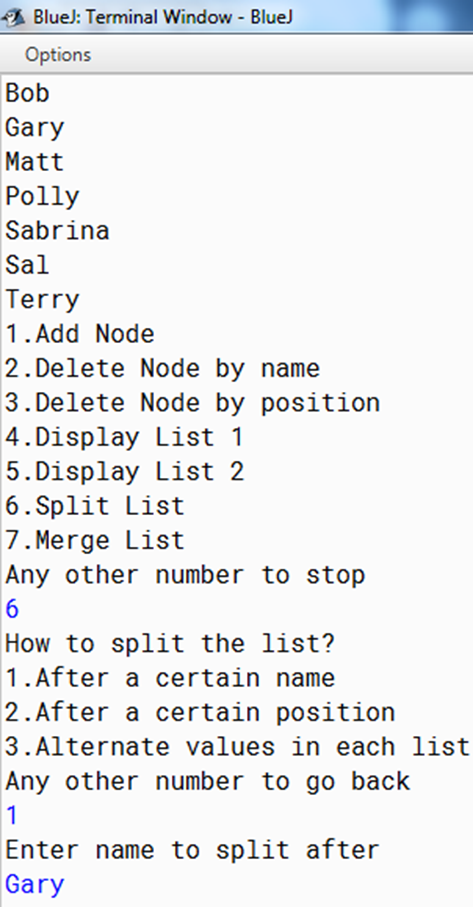
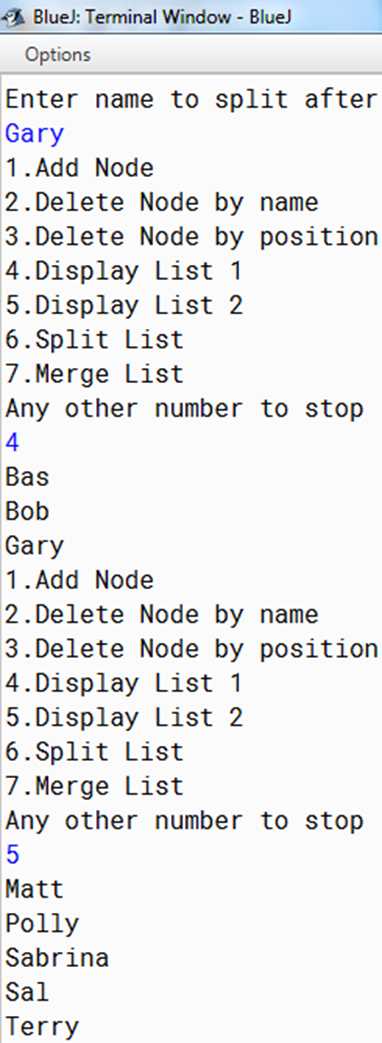
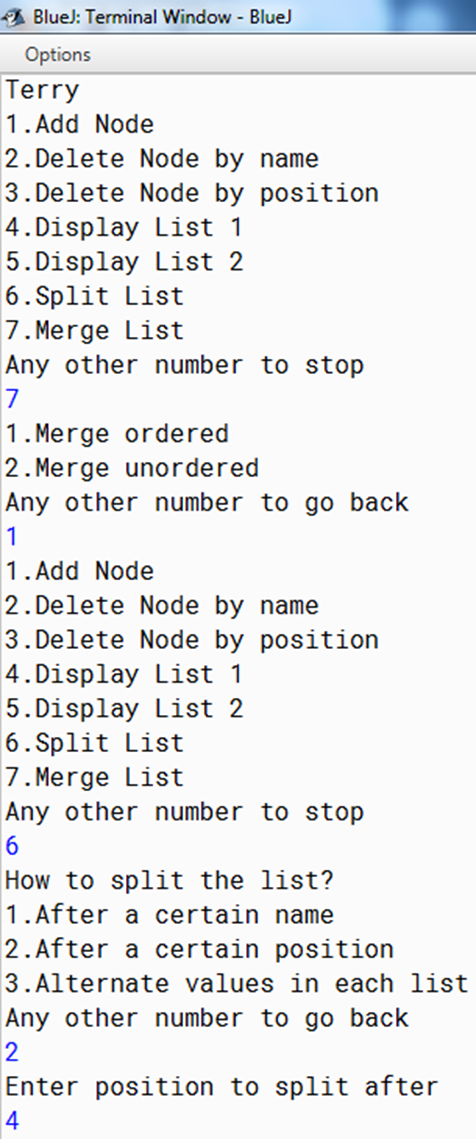
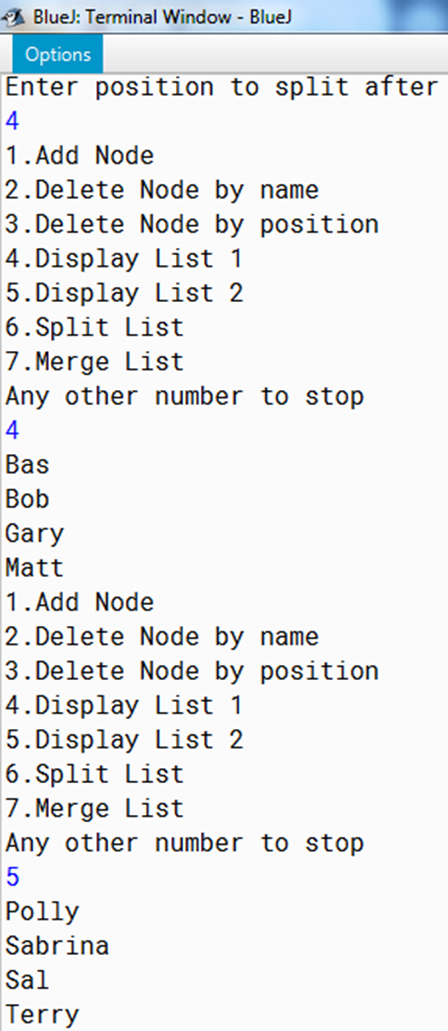
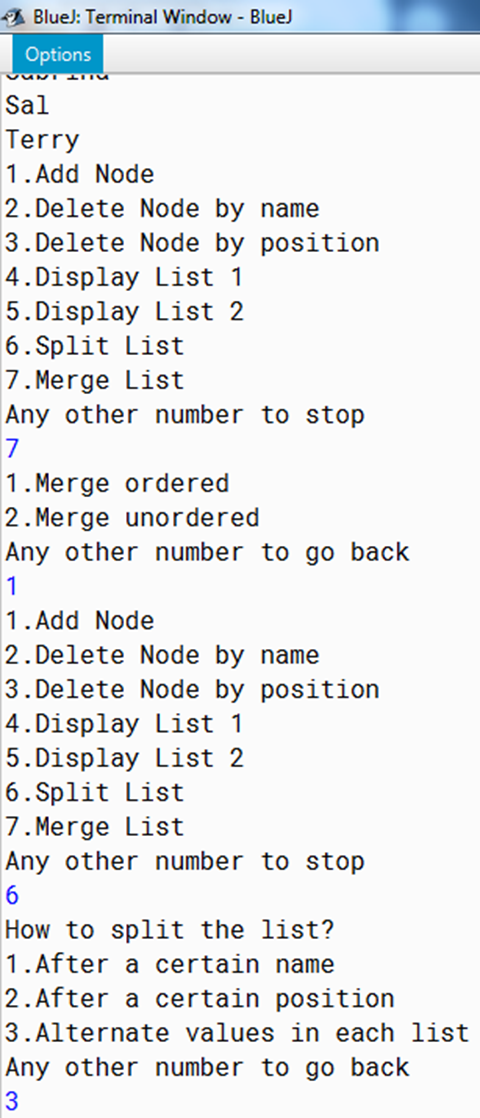
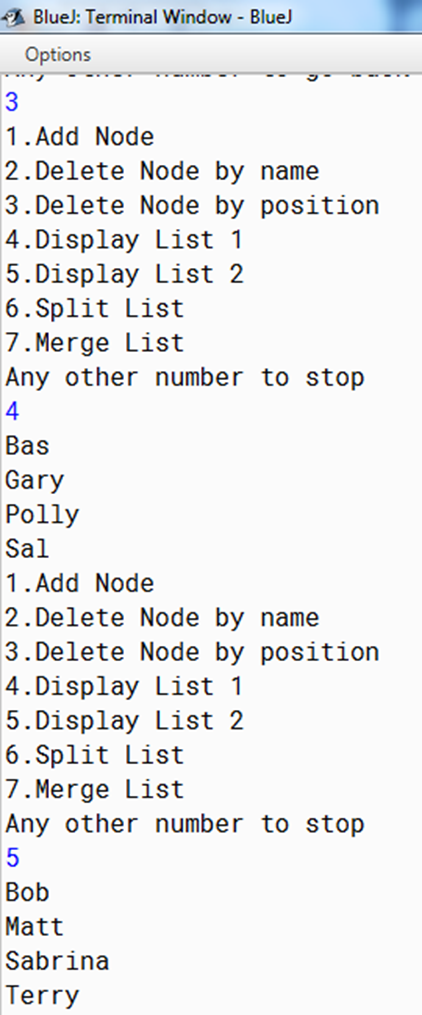
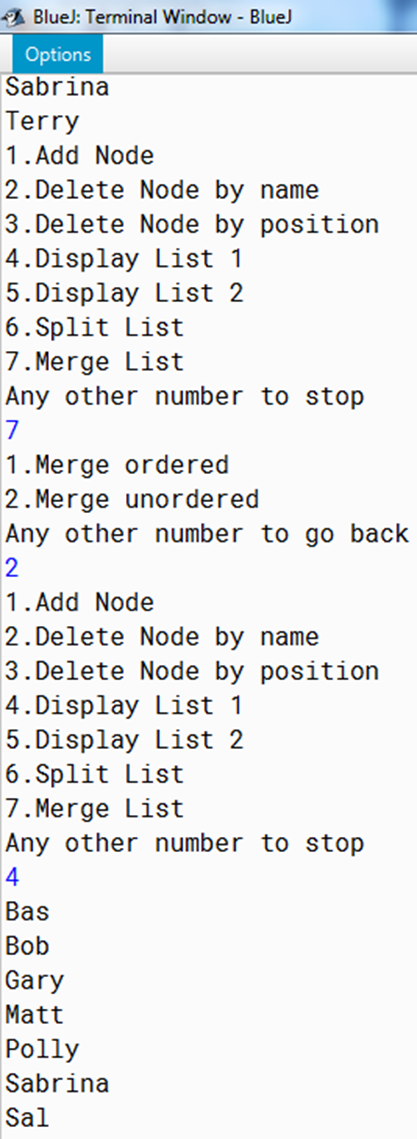
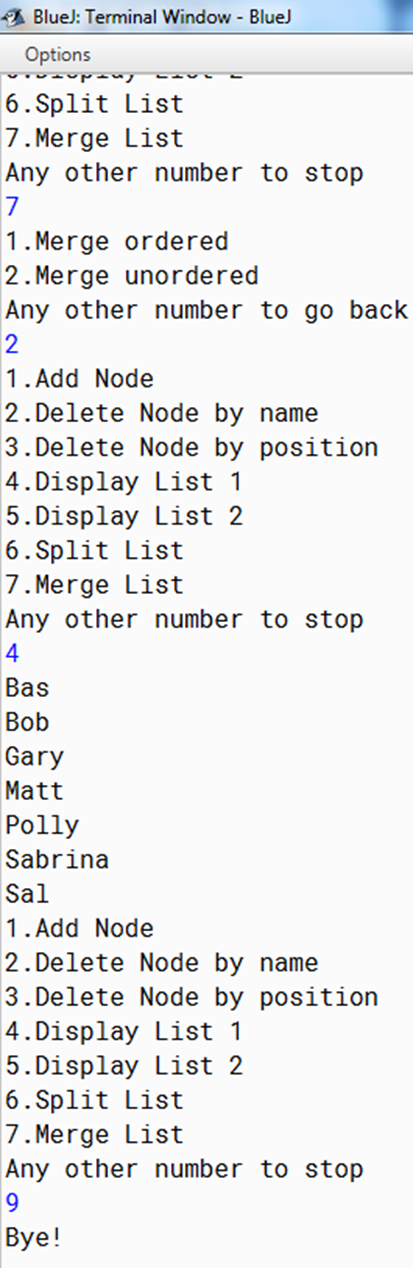
20.Composite Magic Numbers
import java.util.*;
class CompositeMagicNumbers
{
boolean composite(int x)
{
int count=0;
for (int i=2;i<x/2;i++)//checking if it has more than 1 factor
{
if (x%i==0)
count++;
}
if (count>=1)
{
return true;
}
else
return false;
}
boolean magic(int x)
{
int sum=0, temp=0;
while (x>=10)
{
temp=x;
sum=0;
while (temp>0)
{
sum=sum+(temp%10);
temp=temp/10;
}
x=sum;
}
if (x==1)
return true;
else
return false;
}
}
public class CompositeMagicNumbersRun
{
public static void main (String args[])
{
try
{
int count=0,i;
CompositeMagicNumbers a=new CompositeMagicNumbers();
Scanner sc=new Scanner (System.in);
System.out.println("Enter Integer M");
int m=sc.nextInt();
System.out.println("Enter Integer N larger than M");
int n=sc.nextInt();
while (m>n)
{
System.out.println("Re-enter m");
m=sc.nextInt();
}
System.out.println("THE COMPOSITE MAGIC INTEGERS ARE:");
for (i=m;i<=n;i++)
{
if (a.magic(i)==true&&a.composite(i)==true)
{
System.out.print(i+", ");
count++;
}
}
System.out.println();
System.out.println("FREQUENCY OF COMPOSITE MAGIC INTEGERS IS: "+count);
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}

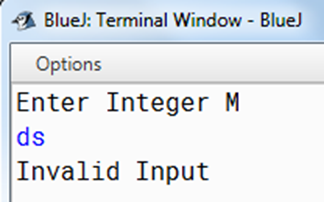
21.Matrix Mirror
//mirrors an inputted matrix
import java.util.*;
public class MatrixMirror
{
int A[][];
int m;
MatrixMirror(int m)
{
this.m=m;
A = new int [m][m];
}
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
int m;
System.out.println("Enter m");
m = sc.nextInt();
if (m>20||m<2)
{
System.out.println("m is an invalid input");
System.exit(0);
}
MatrixMirror a = new MatrixMirror(m);
System.out.println("Enter values for an array");
for (int i = 0;i<m;i++)
{
for (int j = 0;j<m;j++)
{
a.A[i][j] = sc.nextInt();
}
}
a.display(m);
System.out.println("");
System.out.println("");
System.out.println("");
System.out.println("");
a.mirror(m);
a.display(m);
}
void display(int m)
{
for (int i = 0;i<m;i++)
{
for (int j = 0;j<m;j++)
{
System.out.print(A[i][j] + " ");
}
System.out.println(" ");
}
}
void mirror(int m)
{
int B[][] = new int[m][m];
int count = 0;
for (int i = 0;i<m;i++)
{
count = 0;
for (int j =0;j<m;j++)
{
B[i][j] = A[i][m-1-count];
count++;
}
}
for (int i = 0;i<m;i++)
{
for (int j =0;j<m;j++)
{
A[i][j] = B[i][j];
}
}
}
}
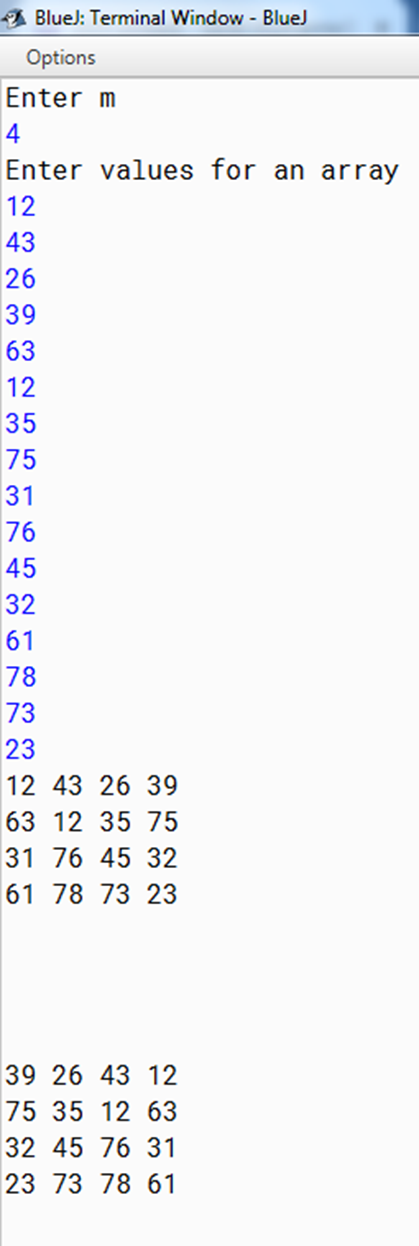
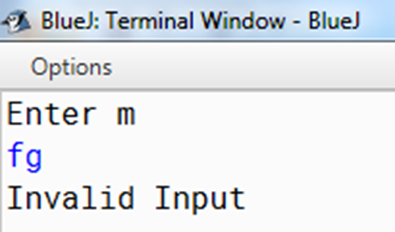
//turns an inputted array into a spiraling matrix
import java.util.*;
public class SpiralArrayClock
{
public static void main (String args[])
{
Scanner scr=new Scanner (System.in);
int count=1, n, i, j, c1=0, r1=0, c2=0, r2=0, flag=0;
System.out.println("Enter n");
n=scr.nextInt();
int arr[][]=new int [n][n];
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
System.out.print(String.format("%4s",count++));
}
System.out.println();
}
count=1;
System.out.println();
System.out.println();
while (count<=n*n)
{
for (i=c1;i<n-c1;i++)
{
arr[c1][i]=count;
count++;
}
c1++;
if (count>n*n)
break;
for (i=1+r1;i<n-r1;i++)
{
arr[i][n-1-r1]=count;
count++;
}
r1++;
if (count>n*n)
break;
for(i=n-2-c2;i>=c2;i--)
{
arr[n-c2-1][i]=count;
count++;
}
c2++;
if (count>n*n)
break;
for (i=n-2-r2;i>r2;i--)
{
arr[i][r2]=count;
count++;
}
r2++;
if (count>n*n)
break;
}
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
System.out.print(String.format("%4s",arr[i][j]));
}
System.out.println();
}
}
}
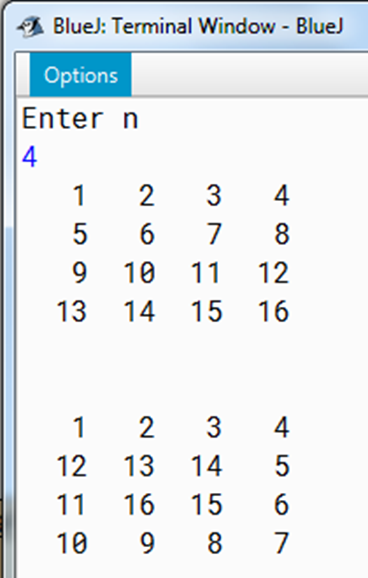
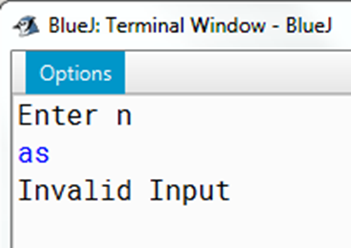
23.Square Rotate
//rotates an inputted square array to the right
import java.util.*;
public class SquareRotate
{
public static void main(String args[])
{
try
{
Scanner scr=new Scanner (System.in);
int n, i, count=0, j;
System.out.println("Enter side of square");
n=scr.nextInt();
int arr[][]=new int [n][n];
System.out.println("Enter the "+(n*n)+" elements of the square");
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
arr[i][j]=scr.nextInt();
}
}
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
System.out.print(String.format("%4s",arr[i][j]));
}
System.out.println();
}
System.out.println();
int temp[]=new int[n*n];
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
temp[count]=arr[i][j];
count++;
}
}
count=0;
for (j=n-1;j>=0;j--)
{
for (i=0;i<n;i++)
{
arr[i][j]=temp[count];
count++;
}
}
System.out.println();
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
System.out.print(String.format("%4s",arr[i][j]));
}
System.out.println();
}
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
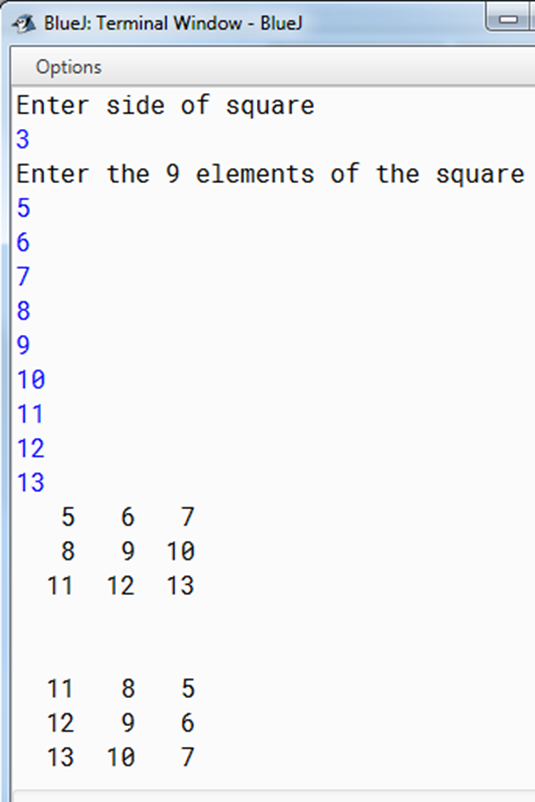
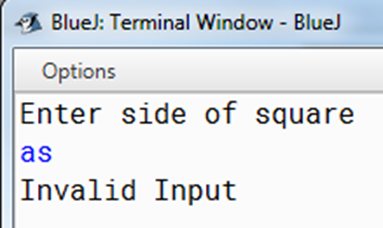
24.Array
//sorts (with bubble sort) and searches an array
import java.util.*;
class myArray
{
int n;
int arr[];
myArray (int size)
{
n=size;
arr=new int [n];
}
void readArray()
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter "+n+" integers");
for(int i=0; i<n;i++)
{
arr[i]=scr.nextInt();
}
}
int Search (int num)
{
int l=0, h=n-1;
int m=l+h/2;
while (l!=h)
{
if (arr[m]==num)
return m+1;
if (num>m)
l=m+1;
else
h=m-1;
m=l+h/2;
}
return -999;
}
void BubSort()
{
int j, i, temp=0;
for(i=0; i<n;i++)
{
for (j=1;j<n-i;j++)
{
if (arr[j-1]>arr[j])
{
temp=arr[j-1];
arr[j-1]=arr[j];
arr[j]=temp;
}
}
}
}
void displayArray()
{
for(int i=0; i<n;i++)
{
System.out.print(arr[i]+",");
}
System.out.println();
}
}
public class myArrayRun
{
public static void main (String args[])
{
try
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter size of the array");
int x=scr.nextInt();
myArray a=new myArray(x);
a.readArray();
a.displayArray();
a.BubSort();
a.displayArray();
System.out.println("Enter search variable");
x=scr.nextInt();
System.out.println("Found at position "+a.Search(x));
System.out.println();
System.out.println("Enter search variable");
x=scr.nextInt();
System.out.println("Found at position "+a.Search(x));
System.out.println();
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
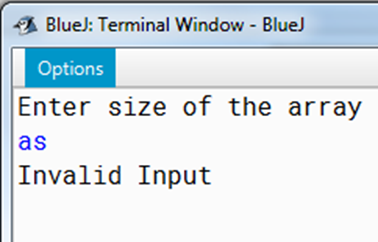
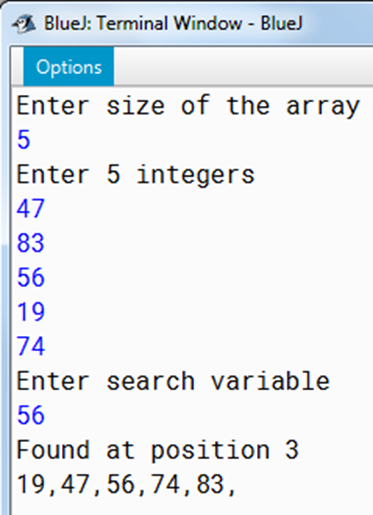
//sorts an array of employee codes and binary searches an inputted code.
import java.util.*;
class indexedarr
{
int arr[]=new int [5];
indexedarr()
{
for (int i=0;i<5;i++)
{
arr[i]=0;
}
}
void fillarr()
{
Scanner scr=new Scanner (System.in);
for (int i=0;i<5;i++)
{
System.out.println("Enter the "+(i+1)+"th employee code");
arr[i]=scr.nextInt();
}
}
void sortarr()
{
int j, i, temp, m;
for (i=0;i<5;i++)
{
if (i!=0)
{
temp=arr[i];
m=i;
for (j=i;j<5;j++)
{
if (arr[j]<temp)
{
temp=arr[j];
m=j;
}
}
temp=arr[i];
arr[i]=arr[m];
arr[m]=temp;
}
}
}
int binarysearch (int b)
{
int l=0, m, h=4;
while (l!=h)
{
m=(l+h)/2;
if (arr[m]==b)
return m;
if (b>arr[m])
l=m+1;
else
h=m-1;
}
return 0;
}
}
public class indexedarray
{
public static void main (String args[])
{
try
{
indexedarr iar= new indexedarr();
iar.fillarr();
iar.sortarr();
for (int i=0;i<5;i++)
System.out.println((i+1)+"= "+iar.arr[i]);
System.out.println();
Scanner scr=new Scanner (System.in);
int p;
System.out.println("Enter a search employee code");
p=scr.nextInt();
if(iar.binarysearch(p)==0)
System.out.println("Not found");
else
System.out.println("Found in position "+(iar.binarysearch(p)+1));
}
catch(Exception a)
{
System.out.println("Invalid Input");
}
}
}
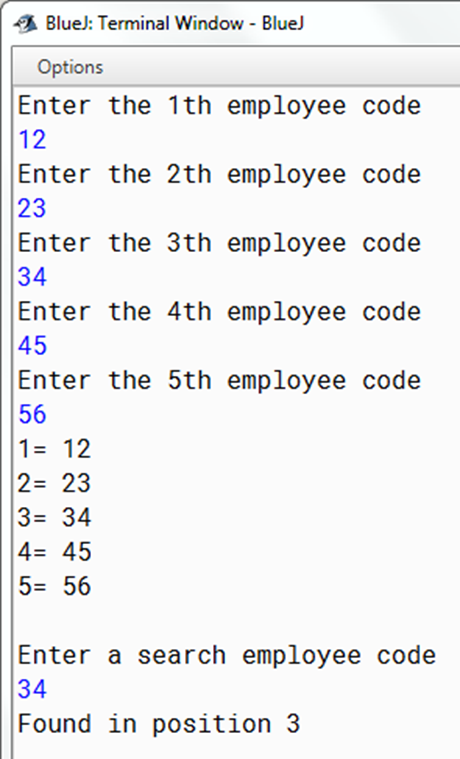
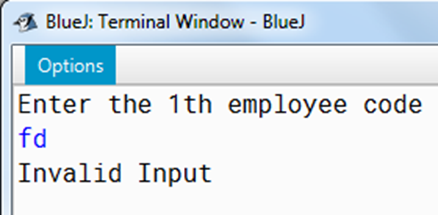
//creates a binary tree from inputted integers and outputs in Pre-order, Post-order and In-order
import java.util.*;
class Node
{
Node l,r;
int d;
Node ()
{
l=r=null;
}
}
class BinaryTree
{
Node root;
BinaryTree ()
{
root=null;
}
Node addNode(Node p, int d)
{
if (p==null)
{
p=new Node();
p.d=d;
}
else
{
if (p.d<d)
p.r=addNode(p.r,d);
if (p.d>d)
p.l=addNode(p.l,d);
}
return p;
}
void InOrder(Node p)
{
if (p.l!=null)
{
InOrder(p.l);
}
System.out.print(p.d+" ");
if (p.r!=null)
{
InOrder(p.r);
}
}
void PreOrder(Node p)
{
System.out.print(p.d+" ");
if (p.l!=null)
{
PreOrder(p.l);
}
if (p.r!=null)
{
PreOrder(p.r);
}
}
void PostOrder(Node p)
{
if (p.l!=null)
{
PostOrder(p.l);
}
if (p.r!=null)
{
PostOrder(p.r);
}
System.out.print(p.d+" ");
}
}
public class BinaryTreeRun
{
public static void main (String args[])
{
try
{
Scanner scr=new Scanner (System.in);
BinaryTree t=new BinaryTree();
System.out.println("Enter integers");
for (int i=0; i<=6; i++)
{
int d=scr.nextInt();
t.root=t.addNode(t.root, d);
}
System.out.print("In-order: ");
t.InOrder(t.root);
System.out.println();
System.out.print("Pre-Order: ");
t.PreOrder(t.root);
System.out.println();
System.out.print("Post-order: ");
t.PostOrder(t.root);
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
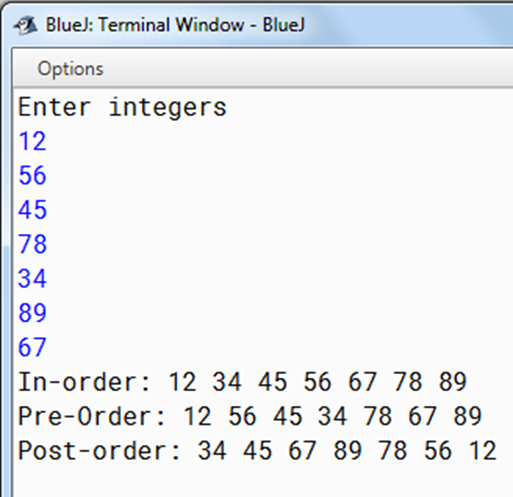
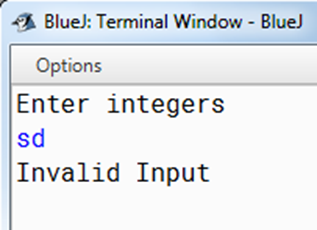
27.Wondrous Square
//determines if an inputted square is wondrous, and gives locations of all prime numbers in it.
//wondrous square is an n by n grid which fulfils the following conditions:
//(i) It contains integers from 1 to n2, where each integer appears only once.
//(ii) The sum of integers in any row or column must add up to 0.5 * n * (n2 + 1).
import java.util.*;
public class WondrousSquare
{
public static void main (String args[])
{
try
{
Scanner scr=new Scanner (System.in);
int n, i, j, k, count=0;
System.out.println("Enter the size of the square, between 2 and 10");
n=scr.nextInt();
while (n<2||n>10)
{
System.out.println("Wrong input, try again");
n=scr.nextInt();
}
int arr[][]=new int [n][n];
System.out.println("Enter the "+(n*n)+" elements of the square");
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
arr[i][j]=scr.nextInt();
}
}
double sval=n*((n*n)+1)*0.5;
sval=(int)sval;
int sum, flag=0, cflag=0;
for (i=0;i<n;i++)
{
sum=0;
for (j=0;j<n;j++)
{
sum=sum+arr[i][j];
}
if (sum!=sval)
flag=1;
}
for (j=0;j<n;j++)
{
sum=0;
for (i=0;i<n;i++)
{
sum=sum+arr[i][j];
}
if (sum!=sval)
flag=1;
}
for (k=1; k<=(n*n);k++)
{
count=0;
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
if (k==arr[i][j])
count++;
}
}
if (count<=1)
cflag=1;
}
if (flag==0&&cflag==1)
System.out.println("YES IT REPRESENTS A WONDROUS SQUARE.");
else
System.out.println("NOT A WONDROUS SQUARE.");
int primen[]=new int [n*n];
int primer[]=new int [n*n];
int primec[]=new int [n*n];
count=0;
for (i=0;i<n;i++)
{
for (j=0;j<n;j++)
{
flag=0;
for (k=2;k<arr[i][j]-1;k++)
{
if (arr[i][j]%k==0)
{
flag++;
break;
}
}
if (flag==0&&arr[i][j]!=1)
{
primen[count]=arr[i][j];
primer[count]=i;
primec[count]=j;
count++;
}
else
{
primen[count]=0;
primer[count]=0;
primec[count]=0;
count++;
}
}
}
int temp, min;
for (i=0;i<n*n-1;i++)
{
min=i;
for (j=i+1;j<n*n;j++)
{
if (primen[j]<primen[min])
min=j;
}
temp=primen[i];
primen[i]=primen[min];
primen[min]=temp;
temp=primer[i];
primer[i]=primer[min];
primer[min]=temp;
temp=primec[i];
primec[i]=primec[min];
primec[min]=temp;
}
System.out.println("PRIME ROW INDEX COLUMN INDEX ");
for (i=0; i<n*n; i++)
{
if (primen[i]!=0)
System.out.println(String.format("%3s%11s%13s",primen[i],primer[i],primec[i]));
}
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
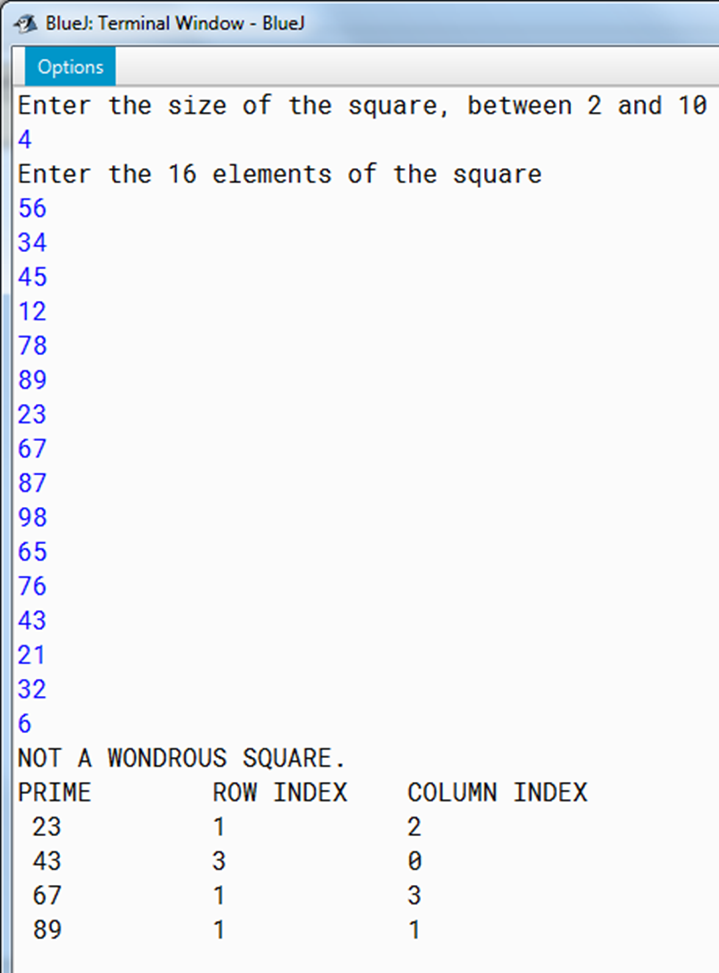
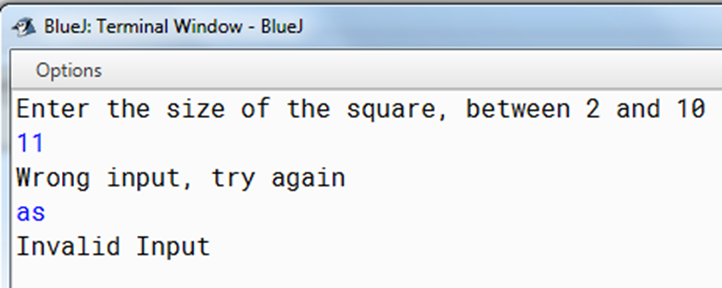
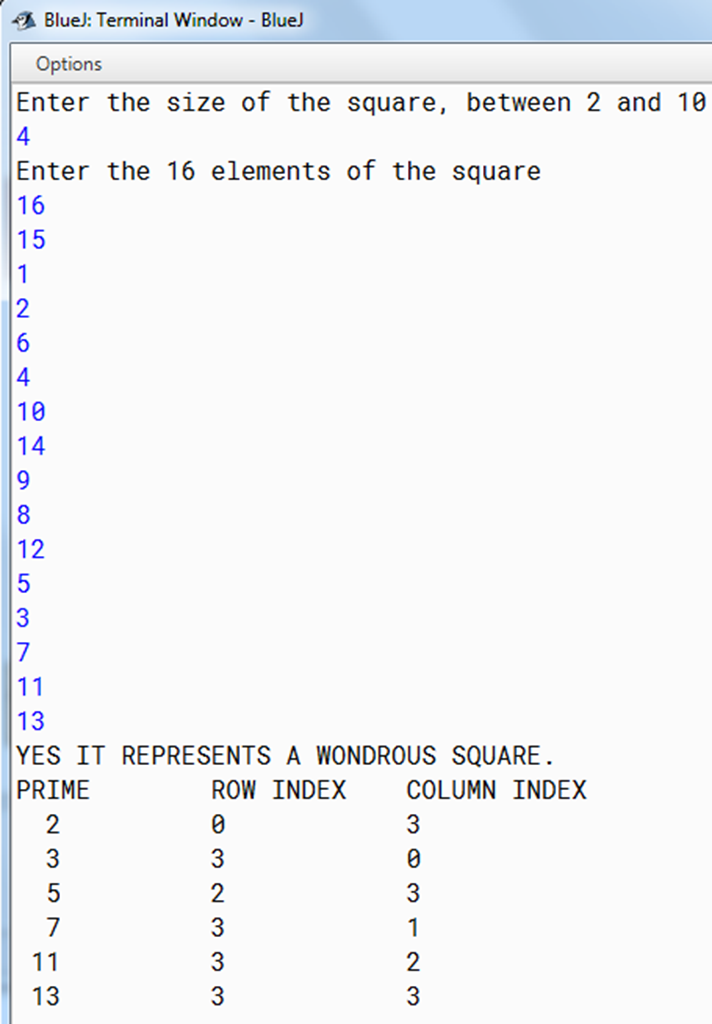
28.Evil Numbers
//checks if a number is an evil number (even number of 1s in the binary expansion)
import java.util.*;
class EvilNumber
{
String toBinary(int n)
{
int r;
String s="";
char dig[]={'0','1'};
while(n>0)
{
r=n%2;
s=dig[r]+s;
n=n/2;
}
return s;
}
int countOne(String s)
{
int c = 0, l = s.length();
char ch;
for(int i=0; i<l; i++)
{
ch=s.charAt(i);
if(ch=='1')
c++;
}
return c;
}
public static void main(String args[])
{
try
{
EvilNumber ob = new EvilNumber();
Scanner sc = new Scanner(System.in);
System.out.print("Enter a positive number : ");
int n = sc.nextInt();
String bin = ob.toBinary(n);
System.out.println("Binary Equivalent = "+bin);
int x = ob.countOne(bin);
System.out.println("Number of Ones = "+x);
if(x%2==0)
System.out.println(n+" is an Evil Number.");
else
System.out.println(n+" is Not an Evil Number.");
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
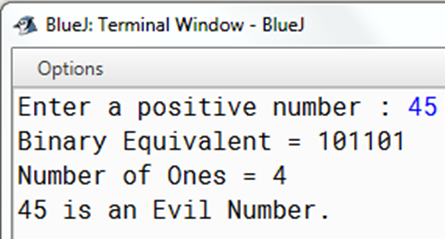
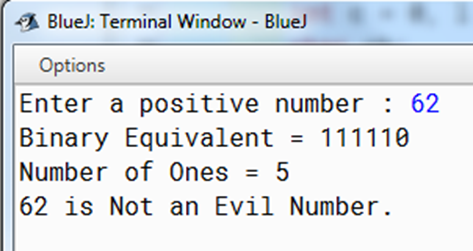
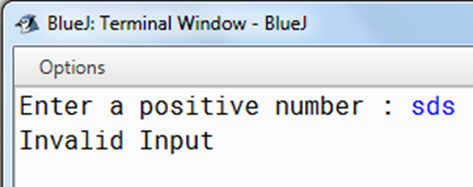
29.Stack
//creates a stack to which numbers can be pushed and popped, displaying over-flow and under-flow.
import java.util.*;
class Stack
{
int stk[], top;
Stack ()
{
top=-1;
}
Stack (int y)
{
stk=new int [y];
}
void push (int x)
{
if (top<stk.length-1)
stk[++top]=x;
else
System.out.println("Stack overflow");
}
int pop ()
{
if (top>0)
return stk[top--];
else
{
System.out.println();
System.out.println("Stack underflow");
return -999;
}
}
}
public class StackRun
{
public static void main()
{
Scanner scr=new Scanner (System.in);
System.out.println("Enter size of stack");
Stack a=new Stack(scr.nextInt());
while (0==0)
{
System.out.println("1.Push\n2.Pop");
int choice=scr.nextInt();
switch (choice)
{
case 1:
System.out.println("Enter number to push");
int x=scr.nextInt();
a.push(x);
break;
case 2:
System.out.println();
System.out.println("Popped :"+a.pop());
System.out.println();
break;
default:
System.exit(0);
}
}
}
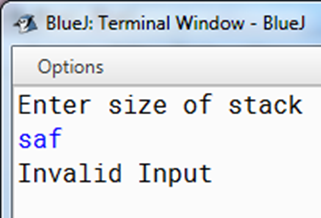
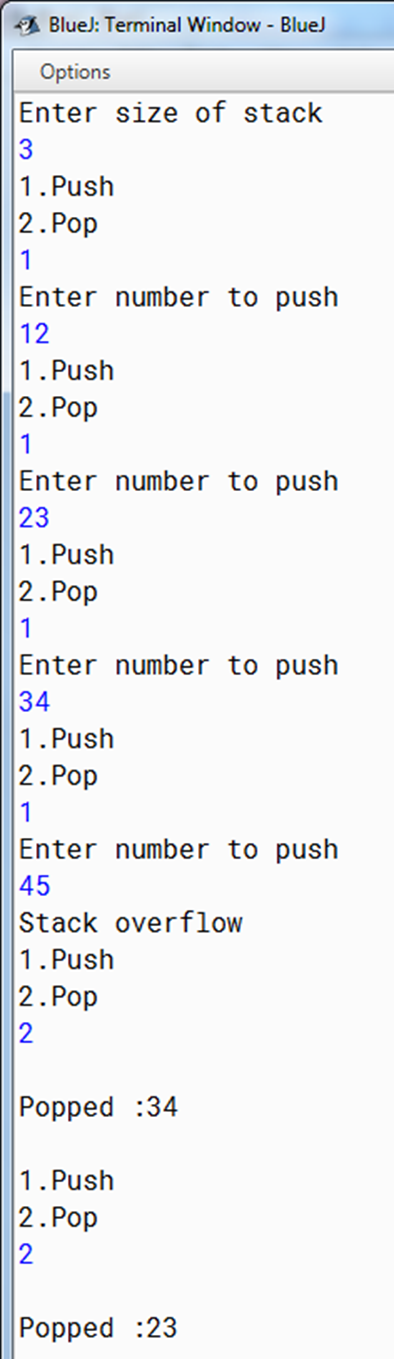
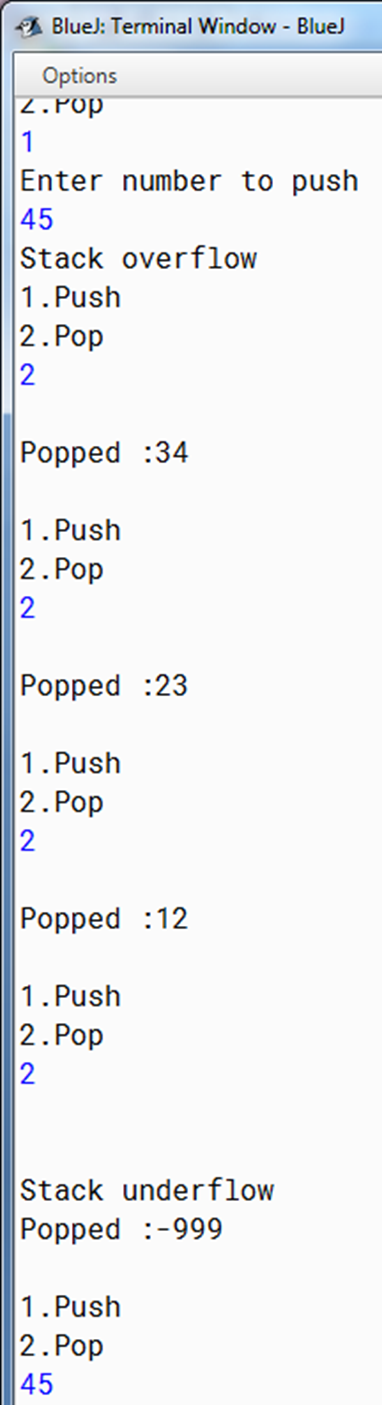
30.Anagrams
//prints all the anagrams of an inputted word and the number of possible anagrams
import java.util.*;
class Anagrams
{
int c = 0;
void input()throws Exception
{
Scanner sc = new Scanner(System.in);
System.out.println("Enter a word");
String s = sc.next();
System.out.print("The Anagrams are: ");
display("",s);
System.out.println("Number of Anagrams = "+c);
}
void display(String s1, String s2)
{
if(s2.length()<=1)
{
c++;
System.out.println(s1+s2);
}
else
{
for(int i=0; i<s2.length(); i++)
{
String x = s2.substring(i, i+1);
String y = s2.substring(0, i);
String z = s2.substring(i+1);
display(s1+x, y+z);
}
}
}
public static void main(String args[])throws Exception
{
try
{
Anagrams a=new Anagrams();
a.input();
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
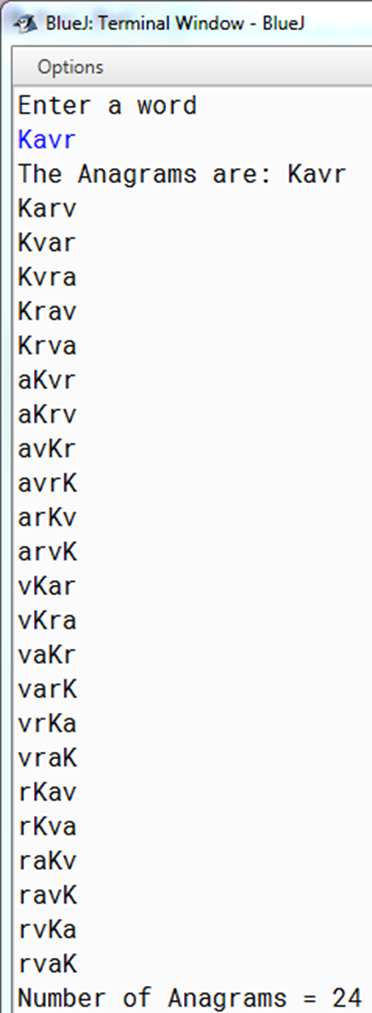
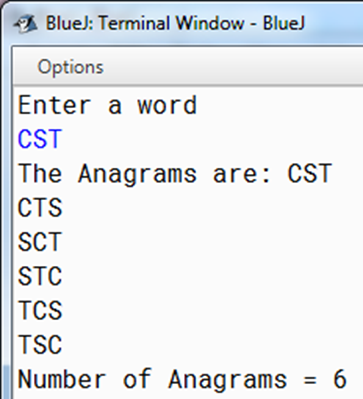
31.Queue
//creates a queue and allows addition and removal of elements, showing queue empty or full
import java.util.*;
class Queue
{
int queue[];
int f,r;
Queue()
{
queue=new int[10];
f=r=-1;
}
void insert(int x)
{
if(f==-1&&r==-1)
{
f=r=0;
queue[r]=x;
}
else if(r>=0&&r<9)
{
r++;
queue[r]=x;
}
else
System.out.println("queue full");
}
int delete()
{
if(f>=0&&f<=r)
{
return queue[f++];
}
else
return -999;
}
public static void main(String args[])
{
try
{
int c=1;
Scanner scr=new Scanner(System.in);
Queue a=new Queue();
while (c!=0)
{
System.out.println("0.Exit");
System.out.println("1.Add");
System.out.println("2.Remove");
c=scr.nextInt();
switch(c)
{
case 1:
System.out.println("Enter number to add");
a.insert(scr.nextInt());
break;
case 2:
int x=a.delete();
if (x==-999)
System.out.println("Queue Empty");
else
System.out.println(x+" Removed");
}
}
}
catch (Exception a)
{
System.out.println("Invalid Input");
}
}
}
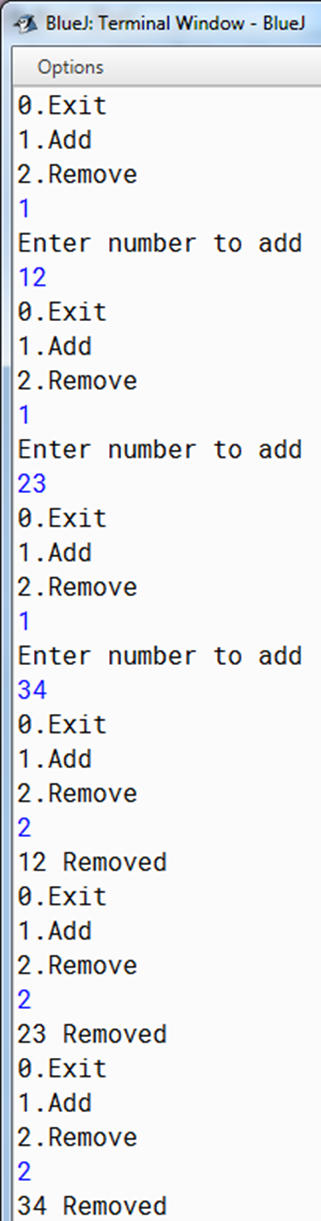
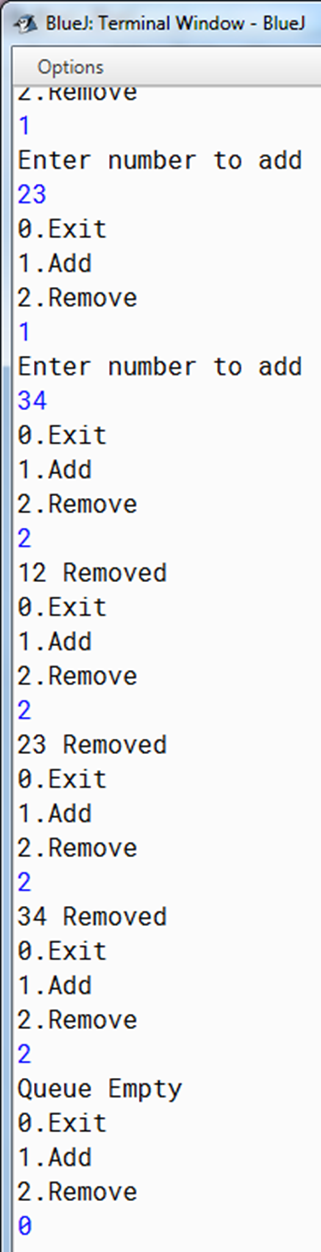